# Recycle the ASP.NET 4 application pool ([ADSI] "IIS://localhost/W3SVC/AppPools/ASP.NET v4.0").psbase.invoke("recycle") # Stop the ASP.NET 4 application pool: ([ADSI] "IIS://localhost/W3SVC/AppPools/ASP.NET v4.0").psbase.invoke("stop") # Start the ASP.NET 4 application pool: ([ADSI] "IIS://localhost/W3SVC/AppPools/ASP.NET v4.0").psbase.invoke("start") # Verify the ASP.NET 4 application pool state: 1 = starting, 2 = started, 3 = stopping, 4 = stopped ([ADSI] "IIS://localhost/W3SVC/AppPools/ASP.NET v4.0").AppPoolStateEnjoy!
Tuesday, October 11, 2011
Using Powershell with Application Pools
While developing Web applications and services using IIS, you need to recycle the application once in a while. You could just run iisreset, but sometimes you only want to affect one specific application pool. If you are using IIS7 or later, you could use appcmd.exe, but if you have some legacy then one option would be powershell. For example, to recycle the ASP.NET 4 application pool using powershell, you can run:
Sunday, October 09, 2011
Avoiding MvcBuildViews build time impact in developers environment by using ASP.NET compiler as an external tool
ASP.NET Razor views (.cshtml) and Web Form views (.aspx) are only compiled by the Web server when they are needed (runtime) and not by Visual Studio when you build your solution (build time). The MvcBuildViews option enables a post-build task (AspNetCompiler Task) to compile your ASP.NET views during build time, thus enabling you to catch syntax errors in the views earlier in the development process. However, enabling MvcBuildViews can significantly increase your build time depending on the size of the Web project you are working on.
To avoid the build time increase, you can enable MvcBuildViews in your build environment only (Release builds) and developers can use the aspnet_compiler.exe as an Visual Studio external tool to precompile ASP.NET views on demand instead of on every build to avoid the building time impact. This way, you will get the best of both worlds: be able to compile views at build time, but not on every build, only when you need it.
To enable MvcBuildViews in release builds only, you can follow the steps described here. To use aspnet_compiler.exe as an external tool in Visual Studio:
- Go to Visual Studio Tools menu, and click on External Tools.
- Enter a title and consider adding a shortcut. I am using Compile ASP.NET &Views and I just use the short cut ALT+T+V to run it.
- Enter the ASP.NET compiler command. For .NET 4, it is C:\WINDOWS\Microsoft.NET\Framework\v4.0.30319\aspnet_compiler.exe.
- Add the following arguments: -c -v temp -p $(ProjectDir) - (option -c forces a full rebuild; option -v defines the virtual path of the application to be compiled, if you use temp, it will be in C:\WINDOWS\Microsoft.NET\Framework\v4.0.30319\Temporary ASP.NET Files\temp; option -p defines the physical path of the application to be compiled, by using $(ProjectDir), it will compile the views of the current project in Visual Studio).
- Enter the initial directory: $(ProjectDir)
- I use the output window, which makes easier if you have errors and want to open the file with errors by double clicking on it.
- Press OK and you are all set. Just make sure to run this command when you are in your ASP.NET web project, or selecting or editing any of its files.
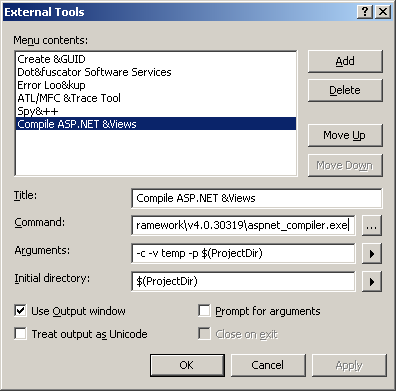
Alternately, you could import the following registry key:
[HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\VisualStudio\10.0\External Tools\Compile ASP.NET &Views] "ToolCmd"="C:\\WINDOWS\\Microsoft.NET\\Framework\\v4.0.30319\\aspnet_compiler.exe" "ToolDir"="$(ProjectDir)" "ToolArg"="-c -v temp -p $(ProjectDir)" "ToolOpt"=dword:00000018
One advantage of using the aspnet_compiler.exe as external tool is to be able to continue work on Visual Studio 2010 while watching the results in the output window. This is way better than using MvcBuildViews in developers build since Visual Studio 2010 hangs and display that annoying message that it is busy.
Happy building!
Subscribe to:
Posts (Atom)
Spring Boot Configuration Properties Localization
Spring Boot allows to externalize application configuration by using properties files or YAML files. Spring Profiles provide a way to segr...
-
I use robocopy to backup my files to a network drive with the following command: robocopy [source folder] [target folder] /MIR The MIR optio...
-
When I try to install WMware Server in Windows 7 (also happened on Vista and XP), I get the message Error 1327 Invalid Drive S:\ and the in...
-
When I created my VMware image, I used only one file for the entire virtual disk (vmdk file). If you want to split your single virtual disk ...